Introduction
I wanted to create some tests for tsParticles (leave a star if you want 🌟, it's free 👀), and I didn't know anything about them.
matteobruni
/
tsparticles
tsParticles - Easily create highly customizable JavaScript particles effects, confetti explosions and fireworks animations and use them as animated backgrounds for your website. Ready to use components available for React.js, Vue.js (2.x and 3.x), Angular, Svelte, jQuery, Preact, Inferno, Solid, Riot and Web Components.
tsParticles - TypeScript Particles
A lightweight TypeScript library for creating particles. Dependency free (*), browser ready and compatible with React.js, Vue.js (2.x and 3.x), Angular, Svelte, jQuery, Preact, Inferno, Riot.js, Solid.js, and Web Components
Table of Contents
-
tsParticles - TypeScript Particles
- Table of Contents
- Do you want to use it on your website?
- Library installation
- Official components for some of the most used frameworks
- Presets
- Templates and Resources
- Demo / Generator
- Video Tutorials
- …
I started searching and I found some informations about Mocha and Chai for writing tests in Javascript and in TypeScript but I had to read multiple articles at once because they were incomplete.
If you need to unit test your TypeScript code you're in the right place.
Let's start!
Requirements
Before starting writing tests we need some libraries added to our project.
I will use npm but you can use yarn too (obvious, thanks).
npm install chai mocha ts-node @types/chai @types/mocha --save-dev
Once the installation is complete we are almost ready.
Add a test
key to your package.json scripts
, like this
"test": "env TS_NODE_COMPILER_OPTIONS='{\"module\": \"commonjs\" }' mocha -r ts-node/register 'tests/**/*.ts'"
This will set the right flag for module, if you have commonjs already you can skip everything until mocha
, for ts-node
that supports only commonjs modules then it runs tests using mocha
.
This is a bit outdated, check out the updated article for a simpler installation.
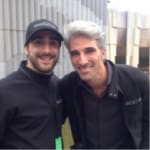
Mocha/Chai with TypeScript (2023 update)
Matteo Bruni ・ Aug 13
I prefer using a tests folder than some .test.ts files. This will require an exclude
key in your tsconfig (if you have one, obviously).
This is a sample taken from my config
"exclude": [
"./tests/",
"./node_modules/",
"./dist/"
],
Once everything is set we can start writing tests.
Tests
Writing a test with chai
is really easy, I started writing almost immediately.
Let's see a sample, it's faster than all words I can write.
import { Options } from '../src/Classes/Options/Options'; // this will be your custom import
import { expect } from 'chai';
describe('Options tests', () => { // the tests container
it('checking default options', () => { // the single test
const options = new Options(); // this will be your class
/* detect retina */
expect(options.detectRetina).to.be.false; // Do I need to explain anything? It's like writing in English!
/* fps limit */
expect(options.fpsLimit).to.equal(30); // As I said 3 lines above
expect(options.interactivity.modes.emitters).to.be.empty; // emitters property is an array and for this test must be empty, this syntax works with strings too
expect(options.particles.color).to.be.an("object").to.have.property("value").to.equal("#fff"); // this is a little more complex, but still really clear
});
});
You can see the simplicity of the syntax, chai
is a really simple test writing library.
If you want to start writing your own tests that's all you need. Super easy.
If you want to read every function available in chai
you can find all of them here.
Running Tests
Once the test is completed we can try it with the command we prepared at the beginning
npm test
and if you did everything right the output will be something like this
> tsparticles@1.13.0-alpha.0 test /path/to/your/project/
> env TS_NODE_COMPILER_OPTIONS='{"module": "commonjs" }' mocha -r ts-node/register 'tests/**/*.ts'
Options tests
✓ checking default options
✓ check default preset options
2 passing (16ms)
If something is not working you'll get a message like this
> tsparticles@1.13.0-alpha.0 test /path/to/your/project/
> env TS_NODE_COMPILER_OPTIONS='{"module": "commonjs" }' mocha -r ts-node/register 'tests/**/*.ts'
Options tests
1) checking default options
✓ check default preset options
1 passing (48ms)
1 failing
1) Options tests
checking default options:
AssertionError: expected undefined not to be undefined
at Context.<anonymous> (tests/Options.ts:19:45)
at processImmediate (internal/timers.js:456:21)
npm ERR! Test failed. See above for more details.
An error blocking any future command, so you can run the tests before the build script, like this
"build": "npm test && tsc",
and with the command below you can now test and build
npm run build
If the tests will pass the build will proceed as expected, otherwise the tests failing will stops the process.
If you want to see my configuration you can find everything here.
Happy Testing 😏
Top comments (14)
Great tutorial, thank you!
I tried for like 3 Hours to test my simple typescript code with karma+jasime (because angular is using it so how hard can it be)...
Looked 2mins on this guide and it works like a charme thank you!
Thanks @matteobruni quite helpful.
I was able to test APIs with minor adjustments.
For context: My source is in
src/ts
including tests undersrc/ts/test
. My compiles produce one js (undersrc/js-tmp
) per source ts (undersrc/ts
). My dists cherry-pick from js-tmp (i.e., exclude tests, ...).`
OMG finally. I tried to add chai testing to my first typescript app instead of karma, all examples inevitable ended with 'Cannot use import statement outside a module'. Tried babel and configured all jsons possible with no luck.
This one finally highlighted the critical part I needed, and because the example is simple and concise it wasn't lost - env TS_NODE_COMPILER_OPTIONS='{"module": "commonjs" }'
many thanks
'env' is not recognized as an internal or external command,
If you are on Windows
env
needs to be replaced withset
, at least this is what I've read googling around.That command is related to
ts-node
as you can read hereYes, but i had to add
&&
.set TS_NODE_COMPILER_OPTIONS={\"module\": \"commonjs\" } && mocha -r ts-node/register 'tests/**/*.ts'
Damn windows, I just wasted the last 24hrs just because of this😩!
Thanks a lot.
I have been using npmjs.com/package/cross-env for cross platform support
Error [ERR_UNSUPPORTED_DIR_IMPORT]: Directory import '/home//node_modules/ts-node/register not supported resolving ES modules imported from /home/mikkel/src//node_modules/mocha/lib/esm-utils.js
Did you mean to import ts-node/register/index.js?
Is pretty much what I get no matter what tutorial I follow.
Thanks, was very helpful on a project of mine.
Is
env TS_NODE_COMPILER_OPTIONS='{\"module\": \"commonjs\" }'
equivalent of adding this to your tsconfig.jsonIt depends if you need that in your tsconfig, which was not my case
Very helpful article.